This plan includes
- Limited free courses access
- Play & Pause Course Videos
- Video Recorded Lectures
- Learn on Mobile/PC/Tablet
- Quizzes and Real Projects
- Lifetime Course Certificate
- Email & Chat Support
What you'll learn?
- 1. Introduction to React
- 2. Data Flow in React
- 3. Component Life-cycle methods
- 4. Components and rendering list
- 5. React Context API
- 6. Working with Forms in React
- 7. Adding Routing using React-Router v4
- 8. State Management with Redux
- 9. Redux thunk and async actions
- 10. Integrating Firebase with React
- 11. Firebase Authentication
Course Overview
React is a JavaScript library for building user interfaces across a variety of platforms. React gives you a powerful mental model to work with and helps you build user inter- faces in a declarative and component-driven way.
Who this course for?
This course is for anyone who’s working on or interested in building user interfaces. Really, it’s is for anyone who’s curious about React, even if you don’t work in UI engineering. You’ll get the most out of this course if you have some experience with using JavaScript to build front-end applications.
Advantages of React:
The following list highlights some of the benefits of React versus other libraries and frameworks:
-
Simpler apps—React has a CBA with pure JavaScript; a declarative style; and pow- erful, developer-friendly DOM abstractions (and not just DOM, but also iOS, Android, and so on).
-
Fast UIs—React provides outstanding performance thanks to its virtual DOM and smart-reconciliation algorithm, which, as a side benefit, lets you perform testing without spinning up (starting) a headless browser.
-
Less code to write—React’s great community and vast ecosystem of components provide developers with a variety of libraries and components. This is important when you’re considering what framework to use for development.
Pre-requisites
- Basic understanding of Javascript and HTML ES6+
- JavaScript knowledge is beneficial but not a must-have
Target Audience
- This course is for anyone who’s working on or interested in building user interfaces. Really, it’s is for anyone who’s curious about React, even if you don’t work in UI engineering. You’ll get the most out of this course if you have some experience with using JavaScript to build front-end applications.
Curriculum 74 Lectures 06:46:11
Section 1 : Getting Started
- Lecture 2 :
- Create React App using create-react-app cli
- Lecture 3 :
- Create elements in react
- Lecture 4 :
- Creating Nested Elements in React
- Lecture 5 :
- How React uses React Elements to Create Virtual DOM
- Lecture 6 :
- What is DOM?
- Lecture 7 :
- What is Virtual DOM
- Lecture 8 :
- What are Components in React
- Lecture 9 :
- Creating Components in React
- Lecture 10 :
- Adding props Validations in react component.
- Lecture 11 :
- Creating Nested components in React
- Lecture 12 :
- Create State in React Component
- Lecture 13 :
- Update State using events and custom methods
- Lecture 14 :
- Iterate Array and render the components
- Lecture 15 :
- Pass function as props from Parent to Child Component
- Lecture 16 :
- Convert React Components to JSX
- Lecture 17 :
- Module Summary
- Lecture 18 :
- Setup React Application
- Lecture 19 :
- Types and Phases of LifeCycle Methods
Section 2 : Data Flow in React Components
- Lecture 1 :
- Module Introduction
- Lecture 2 :
- State in React Component
- Lecture 3 :
- Shallow Merging with setState
- Lecture 4 :
- Use props, PropTypes and defaultProps in React Component
- Lecture 5 :
- Communicate with Parent and Child Component
- Lecture 6 :
- Module Summary
Section 3 : Component LifeCycle Methods in React
- Lecture 1 :
- What are LifeCycle Methods in React Component
- Lecture 2 :
- Types and Phases of LifeCycle Methods
- Lecture 3 :
- LifeCycle Mounting Methods in Action
- Lecture 4 :
- LifeCycle Updating Methods in Action
- Lecture 5 :
- Error Handling with componentDidCatch
Section 4 : Hacker news App - Building Components
- Lecture 1 :
- Setup React Application
- Lecture 2 :
- Add Bootstrap to React Application
- Lecture 3 :
- Create Mock Restful API with Json-Server
- Lecture 4 :
- Send HTTP Requested in React using axios
- Lecture 5 :
- Iterate Array and render the components
- Lecture 6 :
- Add Bootstrap Card to render List Items
- Lecture 7 :
- Creating Header Component
- Lecture 8 :
- Error Handling with Custom ErrorMessage Component
- Lecture 9 :
- Adding Loading Spinner
Section 5 : React Context API
- Lecture 1 :
- Introduction to React Context API
- Lecture 2 :
- React Context API in Action
- Lecture 3 :
- Create Reducer to update the State in React Context
- Lecture 4 :
- Refactoring Context Code to Store
- Lecture 5 :
- Create new Action to Handle Errors
Section 6 : Working with Forms in React
- Lecture 1 :
- Creating Controlled Component
- Lecture 2 :
- Adding State to the Form
- Lecture 3 :
- Save new record by sending Http Request
- Lecture 4 :
- Creating Reusable Component for Input Form Control
- Lecture 5 :
- Adding Form Validations in React and Bootstrap
Section 7 : Add Routing in React using React-Router
- Lecture 1 :
- Add Link Navigations using React-Router
- Lecture 2 :
- Redirect after submitted new Record
- Lecture 3 :
- Creating NotFound Component
- Lecture 4 :
- Creating new Component to Edit the Record
Section 8 : State Management with Redux
- Lecture 1 :
- Setting up Redux into React application
- Lecture 2 :
- Connect React Component to Redux
- Lecture 3 :
- Delete the Record from the ReduxStore
- Lecture 4 :
- Add Record to ReduxStore
Section 9 : Consuming Http Rest API using Async Action Creators
- Lecture 1 :
- Create Async Action to fetch records from the API
- Lecture 2 :
- Create Async Action to delete records from the API
- Lecture 3 :
- Create Async Action to add new Record
- Lecture 4 :
- Async Action to fetch single record
- Lecture 5 :
- Async Action to update the Link
Section 10 : Integrating React and Redux with Firebase
- Lecture 1 :
- Creating Database on Firebase
- Lecture 2 :
- Fetching data from firebase collection in react component
- Lecture 3 :
- Delete document from firebase collection with react
- Lecture 4 :
- Add document in firebase collection with react
- Lecture 5 :
- Update document from firebase collection
Section 11 : Firebase Authentication with React and Redux
- Lecture 1 :
- User Registration in React and Firebase
- Lecture 2 :
- Logout User
- Lecture 3 :
- User Login with Firebase and React
- Lecture 4 :
- Apply Authentication on private Routes
- Lecture 5 :
- Display error notification in React
- Lecture 6 :
- Deploy React Application to Firebase
Our learners work at
Frequently Asked Questions
How do i access the course after purchase?
It's simple. When you sign up, you'll immediately have unlimited viewing of thousands of expert courses, paths to guide your learning, tools to measure your skills and hands-on resources like exercise files. There’s no limit on what you can learn and you can cancel at any time.Are these video based online self-learning courses?
Yes. All of the courses comes with online video based lectures created by certified instructors. Instructors have crafted these courses with a blend of high quality interactive videos, lectures, quizzes & real world projects to give you an indepth knowledge about the topic.Can i play & pause the course as per my convenience?
Yes absolutely & thats one of the advantage of self-paced courses. You can anytime pause or resume the course & come back & forth from one lecture to another lecture, play the videos mulitple times & so on.How do i contact the instructor for any doubts or questions?
Most of these courses have general questions & answers already covered within the course lectures. However, if you need any further help from the instructor, you can use the inbuilt Chat with Instructor option to send a message to an instructor & they will reply you within 24 hours. You can ask as many questions as you want.Do i need a pc to access the course or can i do it on mobile & tablet as well?
Brilliant question? Isn't it? You can access the courses on any device like PC, Mobile, Tablet & even on a smart tv. For mobile & a tablet you can download the Learnfly android or an iOS app. If mobile app is not available in your country, you can access the course directly by visting our website, its fully mobile friendly.Do i get any certificate for the courses?
Yes. Once you complete any course on our platform along with provided assessments by the instructor, you will be eligble to get certificate of course completion.For how long can i access my course on the platform?
You require an active subscription to access courses on our platform. If your subscription is active, you can access any course on our platform with no restrictions.Is there any free trial?
Currently, we do not offer any free trial.Can i cancel anytime?
Yes, you can cancel your subscription at any time. Your subscription will auto-renew until you cancel, but why would you want to?
Instructor
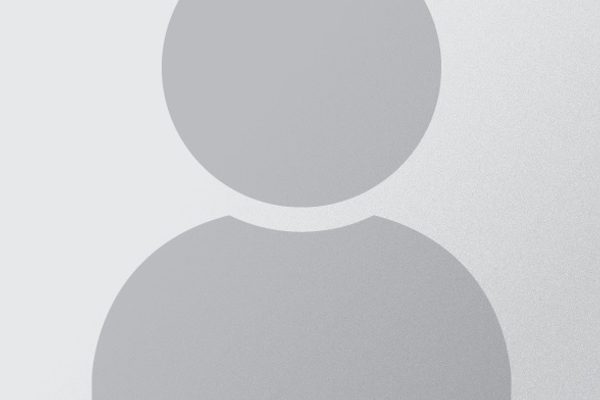
19371 Course Views
2 Courses